The entire purpose of this series to help expose the WordPress Coding Standards, why they matter, and how to write quality WordPress code. In order to do this, we’re taking an in-depth look at each section of the WordPress Coding Standards.
So far, we’ve covered:
- Naming Conventions and Function Arguments
- Single Quotes and Double Quotes
Today, we’re going to be covering the importance of white space. Specifically, we’re going to cover indentation, space usage, and trailing spaces. As easy as it sounds, these are several of the most ignored or misused aspects of the codings standards.
A Word About Whitespace
Before we look at what’s going on with the various coding standards, it’s important to understand why white space is important not only in WordPress, but in programming languages in general.
Simply put, it’s because it enhances readability.
This is why some programming languages are space or tab delimited, this is why some programming languages ask that you space out certain parts of the code such as function parameters, array indexes, and more.
When it comes to WordPress, the conventions are in place not only for readability, but also to provide a consistent reading and development experience for all who work on WordPress themes, plugins, applications, or the core application itself.
Remember: The purpose of coding standards is to make it so that source code looks as if it’s written by a single developer as it sets a level of expectations for contributing developers.
Indentation
As far as indentation is concerned, there’s nothing particularly new, revolutionary, or different as it relates to WordPress. Generally speaking, you’ll indent each time that you start a new block.
This means that:
- Your functions will be indented within the class
- Your conditionals and switch/cases and other blocks will be indented within their functions
- Your loops will be indented within their functions, within their conditionals, and so on
If you’re used to writing code in C-style languages, then there’s nothing particularly new here, right?
An example of what this may look like is exactly this:
1 2 3 4 5 6 7 8 9 10 11 |
function foo( $arguments ) { if ( 0 < count ( $arguments ) { foreach ( $arguments as $arg => $value ) { echo $value ; } } } |
According to the Coding Standards:
Your indentation should always reflect logical structure. Use real tabs and not spaces, as this allows the most flexibility across clients.
The key take aways are that the beginning of lines should begin with tabs, and that the code should reflect a logical structure. Don’t overcomplicate or try to oversimplify things.
There’s one other nuance to white space within the WordPress Coding Standards: Tabs should be used at the beginning of the line, but spaces should be used everywhere else. For the most part, this is an easy rule to follow; however, in WordPress, you’re often going to be creating arrays to pass as arguments.
Ideally, we want each index of the array to line up so that readability is as high as possible but we often have a propensity to use tabs to make it easier to line them up, but this is actually a violation of the coding standards.
1 2 3 4 5 |
$args = array ( 'ID' => 1, 'post_title' => 'The Title' , 'post_content' => 'The content' ); |
As such, make sure that you only use tabs at the beginning of each line.
Space Usage
As it relates to WordPress, I’ve found that spaces have been used much more liberally than in other languages. I’m not saying this simply as an observation, as it was a personal adjustment I needed to make in coming both from .NET and Ruby.
That said, as with most other guidelines in the Codex, there’s nothing that’s particularly complex; however, there are some important distinctions that need to be remembered when writing code.
Spaces should be placed in the following places:
- After commas
- On both sides of logical operators (that is,
||
,&&
, and!
) - On both sides of comparison operators (that is,
<
,>
,==
,===
, etc.) - On both sides of assignment operators (namely
=
) - On both both sides of the opening and closing parenthesis of functions, conditionals, loops, and so on.
- When a variable is being passed as an index of an array, but not when a literal value (such as a string or an integer)
Most of these are relatively straight forward, but in my experience I have found that the array rules tend to cause the most confusion among developers, so here’s a quick note of what’s considered correct and what’s not:
1 2 3 4 5 6 7 |
// Correct spacing... $arr [ $x ] = 'foo' ; $arr [0] = 'bar' ; // Incorrect spacing... $arr [ $x ] = 'bar' ; $arr [ 0 ] = 'bar' ; |
As you can see, it’s nothing terribly complicated but it’s a relatively easy convention to miss.
Trailing Spaces
This rule is likely the easiest standard to remember of them all. Simply put, there should be no trailing spaces left at the end of any line of code.
Depending on your IDE, you may have this filter built in; other times, you may need to install a plugin that will perform this action for you whenever you save the file. But whatever the case, it’s a relatively easy feature to integrate in whatever IDE you’re using.
Additionally, most editors – regardless of if it’s a glorified text editor or a full on IDE – you should have the ability to enable the display of tabs and spaces.
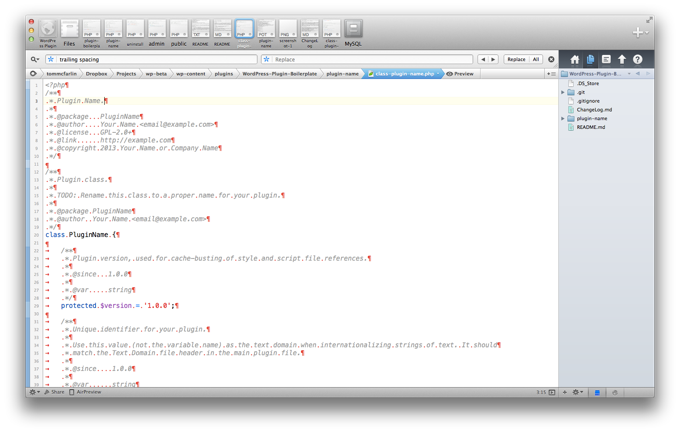
Again, this is one of the simplest conventions to follow and it’s likely that your IDE or editor of choice has this feature or a plugin available that will allow you to automatically do this.
If not, you should be able to activate the ability to see tabs, spaces, carriage returns, and so on so that you can easily identify where the trailing spaces are.
Conclusion
Obviously, white space places a significant role in writing WordPress-based code. In this article, we’ve explored indentation, tabs, spaces, trailing spaces, and why and how to incorporate them into our projects.
In the next article, we’re going to continue talking about the subtleties of the WordPress Coding Standards by looking at brace style, regular expressions, and the nuances of using PHP tags through our WordPress code.